Overview
Hi, I am Joshua and welcome to my Project Portfolio. Office Assistance Specialized Integrated System (OASIS) is one of the projects I have done in my 2nd year studying Computer Science in NUS. OASIS was built up by enhancing, improving and morphing an existing AddressBook application. This project is written in Java and has about 12 kLoC.
OASIS is an employee management application catered for small-to-medium sized enterprises. With the use of primarily textual commands coupled with a beautiful user interface, you can get your administrative jobs done faster than with traditional point-and-click applications. OASIS comes with many efficient problem-solving features that solve employee management problems of today, some of these features are: employee records management, authentication and permissions, assignment management, archiving of employees, and leave management.
My team consists of 4 other year 2 Computer Science students taking CS2103T and this document summarizes my contribution of work done in OASIS.
Summary of contributions
This section shows in detail my contributions to the project, namely my major enhancement, my minor enhancement and other notable contributions to OASIS.
-
Code contributed: see on Reposense
-
Major enhancement: Added the ability to archive and restore employees
-
Added the ability to delete active employees and archive them
-
Behaviour: Allows a user with
DELETE_EMPLOYEE
permission to delete an active employee and send it to the archive list. -
Justification: This feature improves OASIS significantly as it mimics the scenario when an employee leaves the company but data of the employee must be kept for future references, which is an important aspect of employee management.
-
-
Added the ability to restore employees
-
Behaviour: Allows a user with
RESTORE_EMPLOYEE
permission to restore an archived employee and send it to the active list. -
Justification: This feature improves OASIS significantly as it allows rehired ex-employees to be added back to the active employee list with all their past information.
-
-
Added the ability to permanently delete archived employees
-
Behaviour: Allows a user with
DELETE_EMPLOYEE
permission to permanently remove archived employee from the application. -
Justification: This feature improves OASIS significantly as it removes unwanted employee information when they leave the company or when their data is no longer needed.
-
-
Highlights
-
This feature required enhancements to the 4 major components of the application, namely: Logic, Model, Storage and UI. Implementing the feature was challenging as an in depth knowledge of the entire project design must be needed to achieve success.
-
-
-
Minor enhancements:
-
Added list picker in UI which allows the user to change data shown in person list panel by just clicking on the list picker.
-
-
Other contributions:
-
Project management:
-
Managed releases
v1.1
-v1.4
(4 releases) on GitHub
-
-
Enhancements to existing features:
-
Updated the GUI to show a ListPicker to toggle between active and archive list. (Pull requests #115, #174, #178)
-
Updated DeleteCommand to delete from either active or archive list depending on which list the user is on. (Pull requests #178, #183)
-
Updated ClearCommand to clear all employees in OASIS, both active and archive. (Pull requests #183)
-
Wrote tests for features I implemented. (Pull request #183)
-
-
Documentation:
-
Updated the Model class diagram and UI class diagram in the Developer Guide to reflect all the changes that we did.
-
Added documentation for Archive and Restore features in the Developer Guide and User Guide.
-
-
Community:
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
To view deleted employee archive : archive
Displays all archived employees in OASIS.
Archived employees will remain in archive list until they are restored which will send it back to the active list or deleted from archive which will erase all selected employee data completely. |
Format: archive
Type archive
command in box at the top and hit enter and you will be shown the following screen:
To restore employee to active list : restore
Adds the restored employee to the active list and removes the employee from the archive list.
Format: restore INDEX
Examples:
-
restore 2
to delete second employee from the archive list.
Sample output when restore 2
command is executed shown below:
-
Step 1: Type
archive
command in the command box on the top of the application and click enter. -
Step 2: Type
restore 2
command in the same command box as Step 1 and employee at location 2 will be removed from the archive list. You will see the following output on your screen:
-
Step 3: Type
active
command to go back to the active list. Employee restored in Step 2 is now back in the active list as shown below:
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Archive employee feature
Employees with the "DELETE_EMPLOYEE" permissions can delete employees in the system - related to firing employees in real life. Deleted employees from the active list will be moved to the archived list. This feature allows an employee to view the archive list of employees.
Proposed implementation
The archive employee feature is facilitated by VersionedArchiveList
, VersionedAddressBook
and UniquePersonList
.
Given below is an example usage scenario and how the VersionedArchiveList
, VersionedAddressBook
and UniquePersonList
behaves at each step.
Step 1. The user launches the application for the first time. The VersionedArchiveList
and VerisionedAddressBook
will be initialized with the initial archive list and address book state. The UniquePersonList
in both classes will be populated with data from read by storage.
Step 2. The user executes delete 3 command on the active list to delete the 3rd person in the active list. The selected Person object from the UniquePersonList
in VersionedAddressBook
will be transferred to the UniquePersonList
in VersionedArchiveList
and store the deleted Person’s data.
Step 3. The user executes archive command to change view from active list to the archive list.
Step 4. OASIS shows delete person in step 2 in the archive list on the left panel.
The following activity diagram summarizes what happens when a user executes delete and restore command:
Changes to architecture
This section documents the changes made to the architecture and its components.
Aspect: Model
Added a VersionedArchiveList
object. UniquePersonList
in VersionedArchiveList
will store 0 or more Person
objects.
The following diagram shows the class VersionedArchiveList
added to reflect the changes in the Model component:
Aspect: Storage
Added ArchiveListStorage
interface, XmlArchiveListStorage
and XmlSerializableArchiveList
classes to Storage component.
The following diagram shows the interface and classes added to the Storage component:
Design considerations
This section documents the considerations that were carefully studied for implementing this feature. The selected implementation method is denoted by "(current choice)" .
Aspect: Lifetime of objects in Archive list
-
Alternative 1 (current choice): Deleted permanently after being removed by user again.
-
Pros: Guaranteed no loss of data if an employee is accidentally deleted.
-
Cons: May have performance issues in terms of memory usage as employee records stored a few years back could still be stored.
-
-
Alternative 2: Deleted after a certain number of time has passed.
-
Pros: More efficient memory usage wont store old employee records which could cause high memory usage.
-
Cons: Loss of data possible if an employee is accidentally deleted and not restored right away.
-
Aspect: Data structure to support the archive commands
-
Alternative 1 (current choice): Use a list to store the archived employee objects.
-
Pros: Pros: Easy to implement. Only require small modification in existing classes. Faster access to archive list as the user doesn’t need to search every employee in the system to get the employees archived.
-
Cons: We must maintain a separate list for archived objects.
-
-
Alternative 2: Assign an archive attribute to each employee object in the active list and show only in the active list if archived attribute is false. In contrast show in the archive display list if archive attribute is true.
-
Pros: Only need to change 1 attribute when an employee is deleted.
-
Cons: "Archive" is an unusual attribute for a person and it will be time consuming to view the archive list as you must go through all employees to check the archive attribute.
-
Restore employee feature
Employees with the "RESTORE_EMPLOYEE" permissions can restore employees from the archive list into the active list - related to hiring back old employees in real life. The restored employee will be moved back to the active list and removed from the archive list. Employees with “DELETE_EMPLOYEE” permissions can also completely remove employees in the archive list, permanently removing them from OASIS.
Proposed implementation
The Restore or completely remove employee feature is facilitated by VersionedArchiveList
, VersionedAddressBook
and UniquePersonList
.
Given below is an example usage scenario and how the VersionedArchiveList
, VersionedAddressBook
and UniquePersonList
behaves at each step.
Step 1. The user launches the application for the first time. The VersionedArchiveList
and VersionedAddressBook
will be initialized with the initial archive list and address book state. The UniquePersonList
in both classes will be populated with data from read by storage.
Step 2. The user executes archive command to change view from active list to the archive list.
Step 3a1. The user executes restore 1 command to restore the first person in the archive list. The selected Person object from the UniquePersonList
in VersionedArchiveList
will be transferred to the UniquePersonList
in VersionedAddressBook
and be back in the active list.
Step 3a2. The user executes list command to change view from archive list to active list.
Step 3a3. The employee restored in Step 3a1 is shown in active list
Step 3b1. The user executes delete 1 command to completely remove the first person in the archive list from OASIS. The selected Person object from the UniquePersonList
in VersionedArchiveList
will be deleted.
Step3b2. Archive list updated and deleted employee is removed.
The following activity diagram summarizes what happens when a user executes remove and restore command:
The following sequence diagram shows the method calls when the restore command is executed:
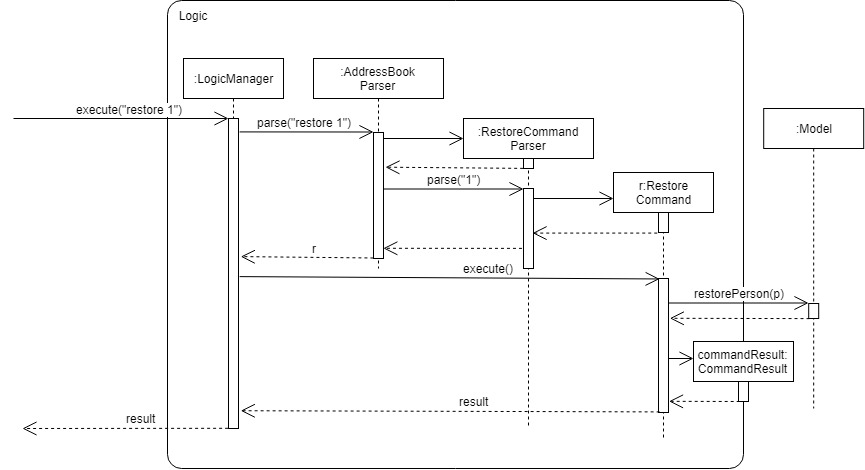
Design considerations
This section documents the considerations that were carefully studied for implementing this feature. The selected implementation method is denoted by "(current choice)" .
Aspect: Data restored from employee
-
Alternative 1 (current choice): All data from the time when the employee was deleted will be restored when the employee object is restored.
-
Pros: Employee being restored wont lose any important information and skips the task of having to key in all the employee’s information again.
-
Cons: Employee may have changed job scopes and permissions assigned may be different, this could lead to big problems if employees have permissions they are not supposed to have.
-
-
Alternative 2: Only certain information like name and phone number will be restored when employee is restored.
-
Pros: More efficient memory usage, don’t have to store other information about the employee. Restored employees will not have the same permissions they had before which could save potential problems of employees having permissions they are not supposed to have.
-
Cons: Could potentially add more workload to the user restoring the employee as the user will need to fill in all necessary details of the employee when the restore command is executed.
-