Overview
OASIS is an employee management application that brings your office together. It is an all-in-one tool for office administration and communication that runs on any computer. With the use of primarily textual commands coupled with a beautiful user interface, you can get your administrative jobs done faster than with traditional point-and-click applications. Some of the main features that you can look forward to using are employee records management, authentication and permissions, assignment management, and leave management.
This portfolio serves as a summary to showcase my contribution for OASIS.
Summary of contributions
The following section shows my various contributions for OASIS.
-
Code contributed: [Link to RepoSense]
-
Major Enhancement: Added Permission System to control what commands each employee can use in OASIS
-
What it does:
-
Ensures that commands are executed only by employees with the permission required for it. It also allow users with the required permission(
ASSIGN_PERMISSION
permission), to view and modify the permissions other employees have.
-
-
Justification:
-
There are many commands in OASIS that should only be performed by employees with certain authorization. Usage of permission allows the admins to easily ensure that previously stated criteria is met by assigning permissions only to authorized employees.
-
-
Highlights:
-
This enhancement requires modification to different components of the application, Model, Storage and Logic. Therefore, implementation was challenging as it requires in-depth knowledge of the different components.
-
This feature is coded to be simple to use and extensible. New permissions can be added simply into
Permission
enum class, and any new Command class only need to 1 line of code to ensure that it can only be executed by authorized employees.
-
-
-
Minor Enhancement: Added Command Auto-Complete feature
-
What it does:
-
When user type in the command box, OASIS will predict what commands the user is going to run, and display a drop down list containing all suggestions.
-
-
Justification:
-
Users might find it hard to memorize commands in OASIS. Having this enhancement will make it easier for them to find the commands they want to execute.
-
-
-
Other contributions
-
Project management:
-
Managed releases
v1.1
-v1.4
(4 releases) on GitHub
-
-
Enhancements to existing features:
-
Community:
-
Documentation:
-
Added documentation for Permission System in the Developer guide.
-
Added documentation for Supporting commands for Permission System Section in the Developer guide
-
Added documentation for Command Auto Complete feature in OASIS at Commands Section in User guide.
-
Added documentation for the following commands in UserGuide. Modify Permission and View Permission.
-
Added Command Summary into User Guide.
-
-
Contributions to the User Guide
The sections shown below are my contribution to the User Guide. They showcase my ability to write documentation for end-users with minimal technical knowledge. |
Command Auto Complete
OASIS has provided you with a command auto complete feature to aid you with the usage of commands. With this functionality, you no longer have to memorise any commands!
When you type commands into the command box, a drop down list of possible commands will appear, as shown in the screenshot below.
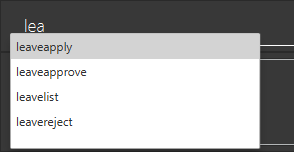
When the drop down list is shown, you can do the following:
-
Use
↑
and↓
to navigate through the list -
Press Enter to select the highlighted option
After selecting the command, you will see that the command will be displayed in the command box.
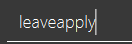
If you wish to see a list of all commands, simply type Space into an empty command box. You should see the list as shown in the screenshot.
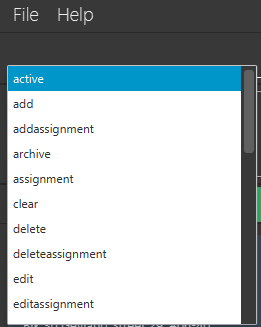
Modify permissions of an emplyee : modifypermission
This command allows you to modify the permissions of an employee.
Format : modifypermission INDEX [-a PERMISSION_TO_ADD]… [-r PERMISSION_TO_REMOVE]…
-
Modifies the permission of the person at the specified INDEX. The index refers to the index number shown in the displayed person list. The index must be a positive integer 1, 2, 3, …
The following is the list of Permissions available.
Permission | What it does |
---|---|
ADD_EMPLOYEE |
Allows the user to add employee |
DELETE_EMPLOYEE |
Allows the user to delete employee |
RESTORE_EMPLOYEE |
Allows user to restore archived employees |
EDIT_EMPLOYEE |
Allows the user to edit information of an employee |
VIEW_EMPLOYEE_LEAVE |
Allows user to view other employee’s applications for leave |
APPROVE_LEAVE |
Allows user to approve and reject leave application |
ADD_ASSIGNMENT |
Allows user to add assignments into OASIS |
DELETE_ASSIGNMENT |
Allows user to delete assignments |
EDIT_ASSIGNMENT |
Allows user to edit assignments |
ASSIGN_PERMISSION |
Allows user to assign permission to employees |
Examples:
-
modifypermission 1 -a ADD_EMPLOYEE
-
modifypermission 2 -a DELETE_EMPLOYEE -r ADD_EMPLOYEE
Contributions to the Developer Guide
The sections shown below are my contribution to the Developer Guide. They showcase both my ability to write technical documentation targeted at future Developers(NUS Computer Science Students) of OASIS, and the technical depth of my contributions to the project. |
Permission System
There are several commands in OASIS that should not be executable by every user. E.g. Add and Delete commands should only be usable by user with the power to hire and dismiss other employees. Permission system is used to ensure that each user are only able to perform commands that they are authorised to when using OASIS.
Current implementation
Aspect: Model
Model of a person have been changed to reflect the permission that each user possesses.
The class highlighted in Red in the following diagram represents the classes that have been created to support the Permission system.
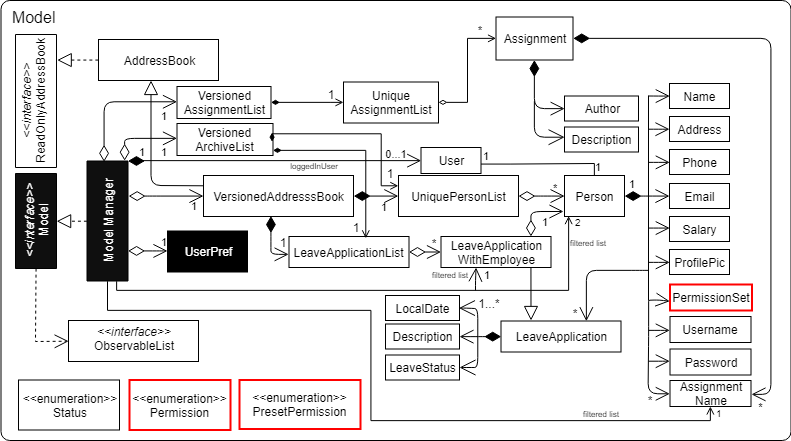
-
Permission
class contains the all possible Permissions that are available to a Person. -
All values in
PermissionSet
must be fromPermission
class. -
PresetPermission
is a enumeration class that resides inPermissionSet
.PresetPermission
is utilised byPermissionSet
class to generate aPermissionSet
object that represents the set of permission that a certain type of User will possess.
As of v1.4, PresetPermission only contains the following preset: Admin , Manager and Employee .
|
Aspect: Storage
Permission have to be stored in the addressbook where the information for Person
is stored. This is achieved through creation of XmlAdaptedPermission
, which was utilised by XmlAdaptedPerson
to store the information in an xml file.
The class highlighted in Red in the following diagram represents the classes that have been created to support the Permission system.
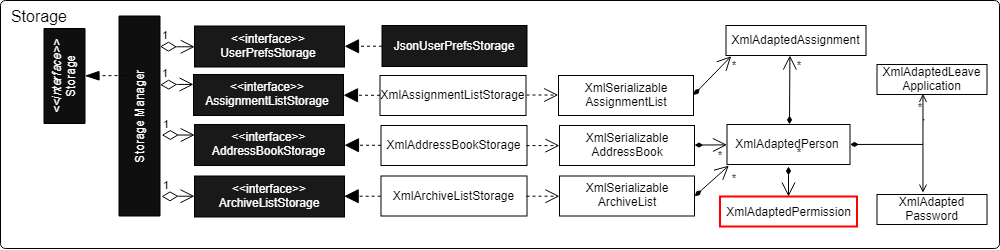
Aspect: Logic
Commands will be required to populate a requiredPermission:PermissionSet
object with all Permission
the command requires user to have to execute the command.
The following is an example on how to assign permission to a Command.
public AddCommand(Person person) {
requireNonNull(person);
requiredPermission.addPermissions(Permission.ADD_EMPLOYEE); (1)
toAdd = person;
}
1 | The method to assign permission to a command |
The code to ensure that each command is only executed by user with the correct permission is located in Command#execute
.
When any command executes, the command will first check if the logged in user possess the correct authorization by comparing requiredPermission
with the user’s permissionSet
object, before performing the command.
Given below is an example scenario of how commands will be executed.
Step 1. The user enters a command delete 1
into the CLI.
Step 2. The system retrieves current user’s PermissionSet
Step 3. The system compares user’s PermissionSet
with DeleteCommand
's requiredPermission
.
-
Two different cases
-
User have required permissions, execute command.
-
User don’t have required permissions, show error message.
-
The following activity diagram summarizes what happens when a user executes a command.
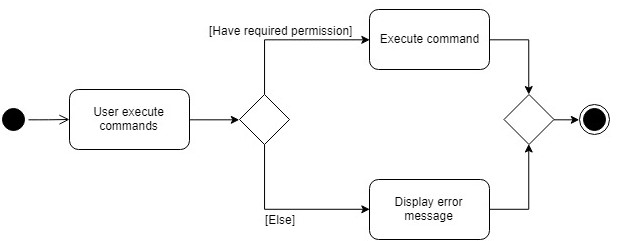
Design Considerations
-
Alternative 1 (Current Implementation): Assign permission to each individual user and restrict commands executable by user based on permission assigned.
-
Pros: Easy to control the commands a user can access.
-
Cons: Need to ensure that there is at least 1 user that can assign permissions to other users. Implementation requires knowledge of multiple components of OASIS.
-
-
Alternative 2: Create subclass of
Person
to be used to identify the role of the user. E.g.Employee
andManager
class. The commands executable by the user will depend on their class.-
Pros: Easy to implement. Only require small modification in existing classes.
-
Cons: Commands cannot be freely assigned to users as it is now dependent on which subclass the user is. E.g. we cannot create an
Employee
with a subset of the commands available toManager
.
-
Supporting commands for Permission System
The following are commands that have been implemented to support the Permission System.
Modify Permissions of employee
This feature allows the user to change the Permission that have been allocated to an employee.
This feature can only be performed by users that have ASSIGN_PERMISSION permission.
|
Current Implementation
This feature allows the user to indicate what permission to add and remove based on the prefix.
-
-a PERMISSION_TO_ADD
to add permission -
-r PERMISSION_TO_REMOVE
to remove permission
Aspect: Logic
To implement this new command syntax, ModifyPermissionCommandParser
utilises ArgumentTokenizer#tokenize
to generate a ArgumentMultiMap
. The ArgumentMultiMap
's key
contains the prefix, and value
contains the list of keywords that succeeded the prefix. There will also be a preamble
which is used to retrieve the index
of the targeted employee.
All the keywords is then added to either permissionToAdd:Set<Permission>
or permissionToRemove:Set<Permission>
depending on their prefix. The 2 sets, together with the index, will be then be used to create ModifyPermissionCommand
.
A ParseException will be thrown if any of the permission names are invalid. |
When ModifyPermissionCommand
is executed, it will then modify the permission of targeted employee, adding permission from permissionToAdd
and removing permissions from permissionToRemove
.
The command will be executed successfully if at least one permission is added or removed. |
The following is a sequence diagram that visualizes how this operation works.
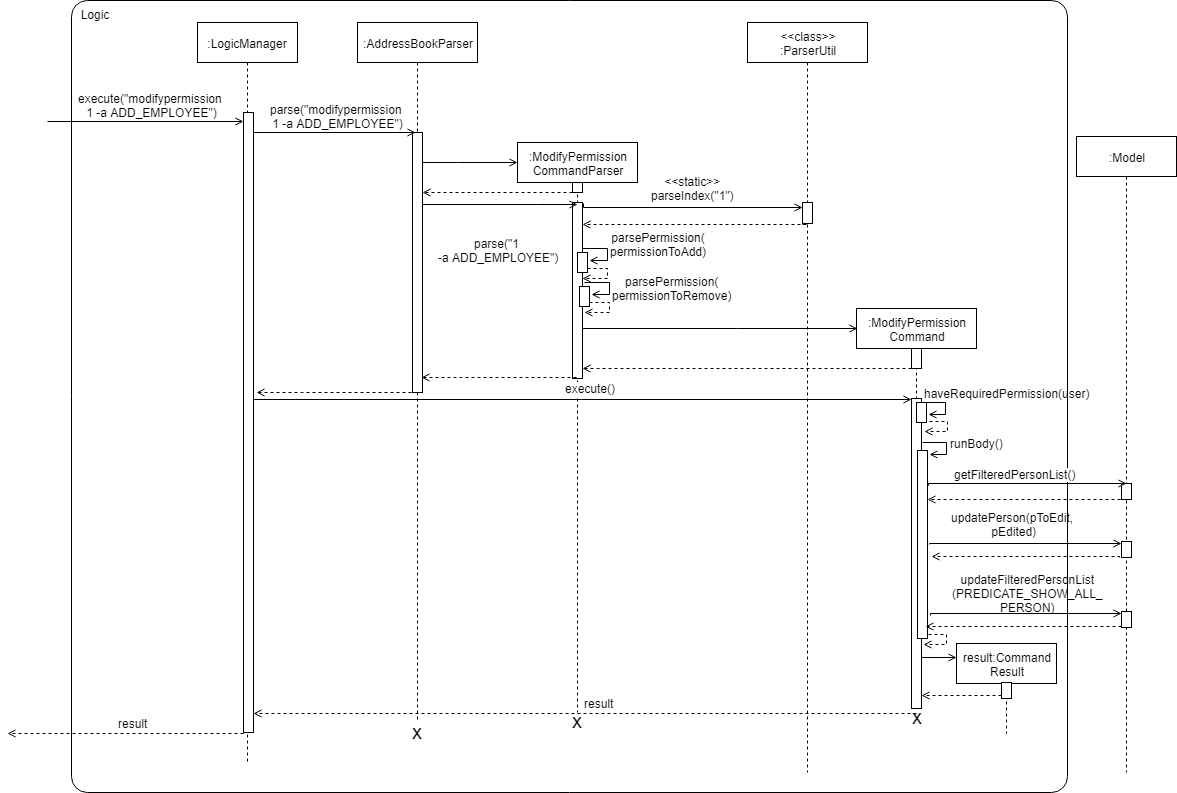
Design Considerations
-
Alternative 1 (Current Implementation): Allow both adding and removing of multiple permissions with one command with the use of prefixes.
-
Pros: Only need to learn how to use 1 command. Can perform both adding and removing of permissions with a single command.
-
Cons: Harder to implement then other alternatives.
-
-
Alternative 2: Create 2 separate commands to handle adding and removing of permission. (E.g.
AddPermissionCommand
andRemovePermissionCommand
)-
Pros: Easy to implement.
-
Cons: User will have to remember 2 commands. In addition to this, User also have to execute at least 2 commands if they wish to both add and remove permission.
-